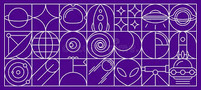
Comparison | Design Patterns ποΈ
Main Design Patterns Design patterns are typical solutions to common problems in software design. They are like pre-made blueprints that one can customize to solve recurring design problems in code. There are 23 classic design patterns defined by the βGang of Fourβ. But some are more frequently used in real-world applications than others. Categories of Design Patterns There are three main categories of design patterns: 1. Creational Patterns 1.1 Singleton Purpose: Ensure a class has only one instance and provide a global point of access to it....
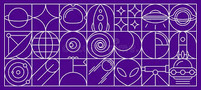
Essentials | Design Patterns Gist π€π€
Main Design Patterns Design patterns are typical solutions to common problems in software design. They are like pre-made blueprints that one can customize to solve recurring design problems in code. There are 23 classic design patterns defined by the βGang of Fourβ. But some are more frequently used in real-world applications than others. Categories of Design Patterns There are three main categories of design patterns: 1. Creational Patterns Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation....
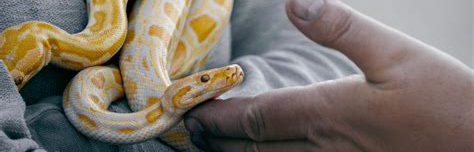
Comprehensive Python Cheatsheet π
Contents 1. Collections: List , Dictionary , Set , Tuple , Range , Enumerate , Iterator , Generator . 2. Types: Type , String , Regular_Exp , Format , Numbers , Combinatorics , Datetime . 3. Syntax: Args , Inline , Import , Decorator , Class , Duck_Types , Enum , Exception . 4. System: Exit , Print , Input , Command_Line_Arguments , Open , Path , OS_Commands ....
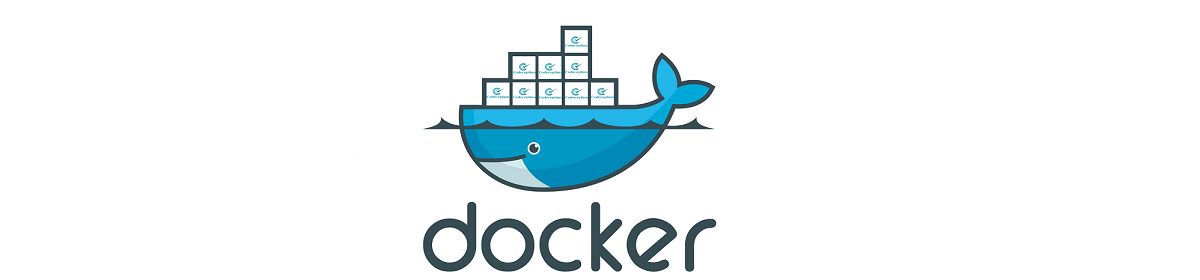
Docker Commands π³
S.No Use Cases Commands 1. See all images present in your local machine docker images 2. Search images in docker-hub (registry) docker search MODULENAME 3. Download image from docker-hub to local machine docker pull MODULENAME 4. Run Docker (Create+Start+Name+Go Inside the Container) docker run -it --name CONTAINERNAME IMAGENAME /bin/bash 5. Check service start or not service docker status OR docker info 6. Start Container docker start CONTAINERNAME 7. Go inside Container docker attach CONTAINERNAME 8....
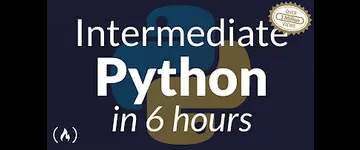
Intermediate Python Codeblocks π
Intermediate Python 1. List Oredered, mutable, allows duplicate items & different data types Creating print("1.1 using [] - list constructor") a1=["banana","cherry","apple"] a2=["banana",2,True,'a'] a3=["a","a"*2,"a"*3] # output: ['a', 'aa', 'aaa'] a20=[20]*10 a21=[1]*10 a22=a20+a21 print(a1,a2,a3,a20,a21,a22,"\n") print('''1.2 using "list()"''') a4=list(a1) a5=list(range(1,6)) a6=list("janav makkar") # output: ['j', 'a', 'n', 'a', 'v', ' ', 'm', 'a', 'k', 'k', 'a', 'r'] a7=list() a8=list(["ab","bc","cd"]) print(a4,a5,a6,a7,a8,"\n") print("1.3 using list comprehensions") a9=[1,2,3,4,5,6,7,8,9,10] a10=[i for i in a9 if i%2==0] a11=[i**2 for i in a9] a12=[i*12 for i in range(11,20)] print(a10, a11, a12,"\n") ### Accessing print("2....
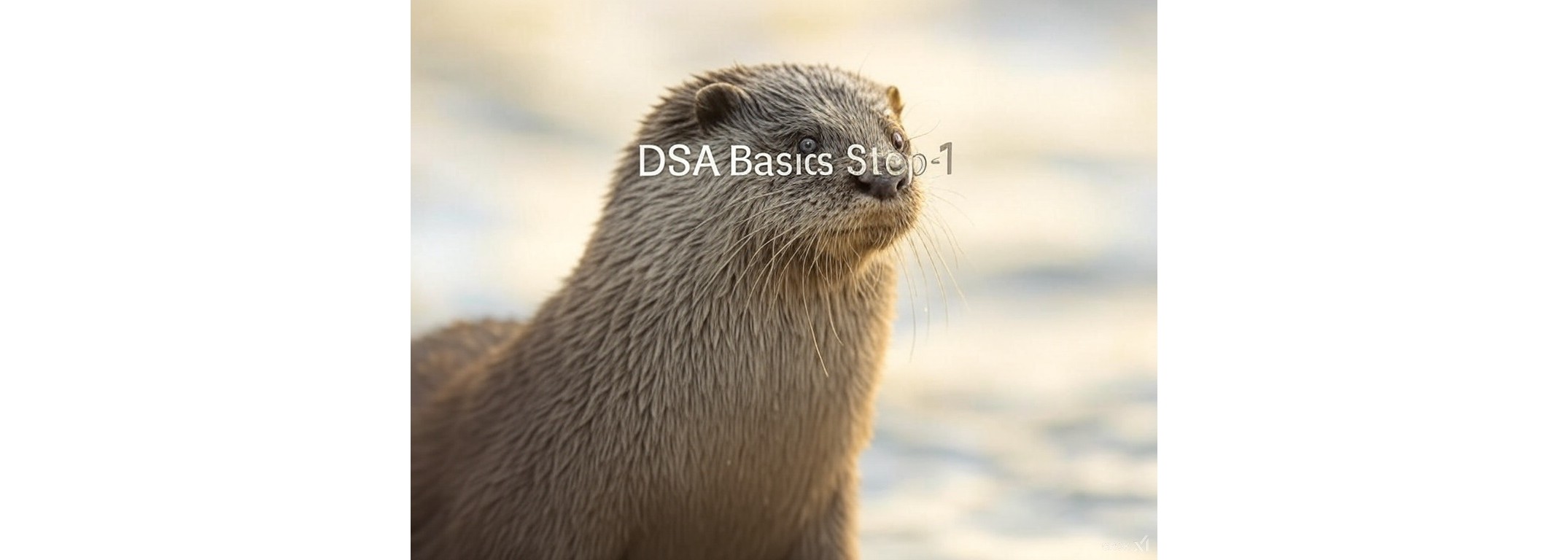
Min Heap Implementation from Scratch | #DSA-450
Min-Heap Min-Heap is a complete binary tree where the parent node is always smaller than or equal to its child nodes. Since a Min Heap is a Complete Binary Tree, it is commonly represented using an array. In an array representation: The root element is stored at Arr[0]. For any i-th node (at Arr[i]): Parent Node β Arr[(i - 1) / 2] Left Child β Arr[(2 * i) + 1] Right Child β Arr[(2 * i) + 2] Operations on Min Heap getMin(): It returns the root element of Min Heap....
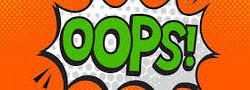
OOPs - 1 | Basic Concepts π¦
1. Class and Object A class is a blueprint for creating objects. It defines a set of attributes and methods that the objects of that class will have. class Dog: def __init__(self, name, breed): self.name = name self.breed = breed def bark(self): return f"{self.name} says Woof!" # Creating an object my_dog = Dog("Buddy", "Golden Retriever") print(my_dog.bark()) # Output: Buddy says Woof! Illustration: Class (Dog) +-------------------+ | Attributes | | - name | | - breed | | | | Methods | | - bark() | +-------------------+ ^ | | Instance | +-------------------+ | Object (my_dog) | | name: Buddy | | breed: Golden | | Retriever | +-------------------+ 2....
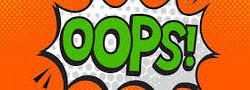
OOPs - 2 | Advanced Tricks in OOPs π¦
1. Property Decorators Properties allow you to use methods like attributes, providing a clean way to implement getters, setters, and deleters. class Temperature: def __init__(self, celsius): self._celsius = celsius @property def celsius(self): return self._celsius @celsius.setter def celsius(self, value): if value < -273.15: raise ValueError("Temperature below absolute zero is not possible") self._celsius = value @property def fahrenheit(self): return (self.celsius * 9/5) + 32 @fahrenheit.setter def fahrenheit(self, value): self.celsius = (value - 32) * 5/9 temp = Temperature(25) print(temp....
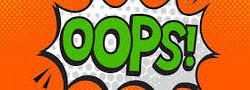
OOPs - 3 | OOPs Application of Advanced OOP Techniques in Programming Challenges π¦
1. Database Connection Pool using Singleton and Context Manager Problem: Managing database connections efficiently in a multi-threaded application. Solution: Use the Singleton pattern to ensure a single connection pool, and a context manager for safe connection handling. import threading from contextlib import contextmanager class DatabasePool: _instance = None _lock = threading.Lock() def __new__(cls): if cls._instance is None: with cls._lock: if cls._instance is None: cls._instance = super().__new__(cls) cls._instance.connections = [] return cls....